Oh noes!
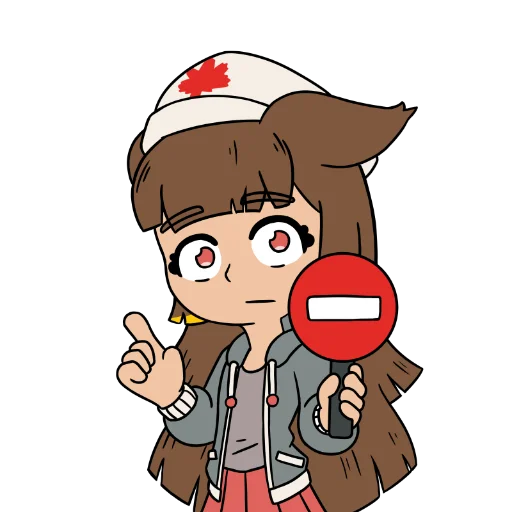
Access Denied: error code 160f2fcf782d9c6db79b1a07eacfaa76543637819f79a02186a502804f259e85.
Go home or if you believe you should not be blocked, please contact the webmaster at contact@costmiku.space
Access Denied: error code 160f2fcf782d9c6db79b1a07eacfaa76543637819f79a02186a502804f259e85.
Go home or if you believe you should not be blocked, please contact the webmaster at contact@costmiku.space